css // Apr 18, 2024
What is a CSS Reset (w/ Examples)?
One of the most important tools for a front-end developer is a CSS reset. A set of pre-defined styles that "reset" the default styles for elements offered by browser vendors.
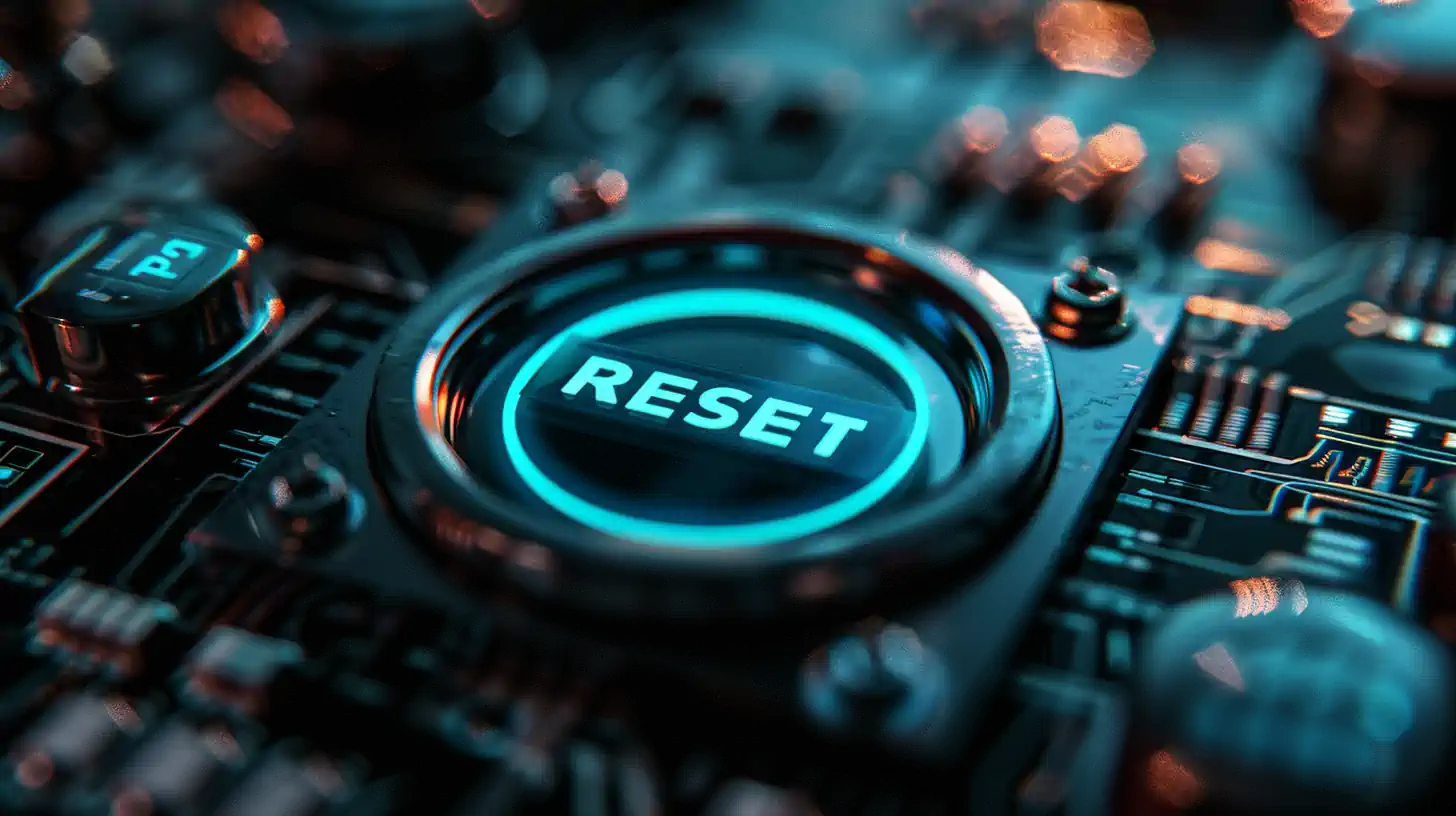
A frustrating reality of web development is dealing with the differences between browser vendors.
While things have improved significantly over the last 15 years, there are still inconsistencies in how browsers implement different parts of the HTML, CSS, and JavaScript specifications.
In particular, all browsers implement a default stylesheet for HTML elements. Because CSS is "cascading" (cascading style sheets) and highly-reactive to specificity in your CSS declarations, it's not uncommon to get a confusing result visually when styling your UI. To get around this, front-end developers came up with the convention of the CSS reset.
Like the name implies, a CSS reset "resets" the default stylesheet for the browser. This removes things like element display
styling, padding, and margin—all of which can conflict with your own, custom stylesheet.
What does a reset look like?
A CSS reset is just like normal CSS but with a specific intent. For example, the following could be considered a reset:
a {
display: block;
}
This is considered a reset because, by default, all <a></a>
tags in your HTML are set to display: inline
.
A more comprehensive CSS reset may look like this:
*,
*:before,
*:after {
position: relative;
box-sizing: border-box;
}
ol,
ul {
padding: 0;
margin: 0;
list-style: none;
}
Again, all we're doing is writing CSS declarations to specify what we want our "blank canvas" to look like.
How a reset works
In terms of specificity, the browser's default styling is loaded first, followed by whatever stylesheets you load into your HTML. When using a reset, it's best to load it as the first stylesheet in your list of custom stylesheets. For example, consider the following <head>
example:
<head>
<title>My App</title>
<style type="text/css">
body { background: #ffffff; }
</style>
<link rel="stylesheet" href="/index.css" />
</head>
Above, we'd expect the rules in the inline <style></style>
tag to be loaded first, followed by the styles inside of the index.css
file loaded beneath it. If we were to work a reset into this, we'd want our HTML to look like this:
<head>
<title>My App</title>
<link rel="stylesheet" href="/reset.css" />
<style type="text/css">
body { background: #ffffff; }
</style>
<link rel="stylesheet" href="/index.css" />
</head>
Here, we'd expect the load order to be:
- The browser's default stylesheet.
- Our
reset.css
file. - The inline style tag.
- The
index.css
file.
Because we put the reset at the top of our own stylesheets, we can trust that our own, custom stylesheets will apply styles "on top" of the CSS provided by the reset. So, in our example reset above, we'd expect all <a></a>
tags to be display: block
and can write our custom CSS accordingly.
What reset should I use?
As you'd expect, there are a lot of different approaches to and opinions about how to author a CSS reset. There's no "right" way as how something looks is highly subjective. What may look good to one developer or designer looks bad to another.
Some developers default to authoring their own reset specific to each project, while others opt for a pre-made, battle-tested reset.
My personal favorite is Eric Meyer's CSS Reset 2.0. I like it because it only strips back browser styling and assumes no other styling. Another popular CSS reset that goes a bit further is Josh Comeau's Modern CSS Reset. That reset goes a bit further and also resets things like typography in the browser, providing a consistent font-size and line-height for text on the page.
As of writing, my own reset is a combination of the Eric Meyer reset along with the following styles:
/* Eric Meyer Reset */
*,
*:before,
*:after {
margin: 0;
padding: 0;
position: relative;
box-sizing: border-box;
min-width: 0;
}
This gets me to a base style where all elements are "flat" (without padding), expand their width to include border, padding, and margin, and ensure that their width doesn't collapse when using display: flex
.
While I don't change this too frequently, as I learn more, I tend to update it across projects to ensure I'm always playing by the same rules (i.e., I don't run into any CSS surprises and can assume my approach to styling remains consistent).
Play around
It's worth setting up a Codepen and testing out a few different resets to see what feels right. For convenience, I've set up a test Codepen using Adam Morse's HTML test file with all HTML elements on one page.