css // Apr 12, 2024
How to Build a Simple Grid Layout with CSS Grid
Using CSS Grid properties to layout a responsive 3x3 grid.
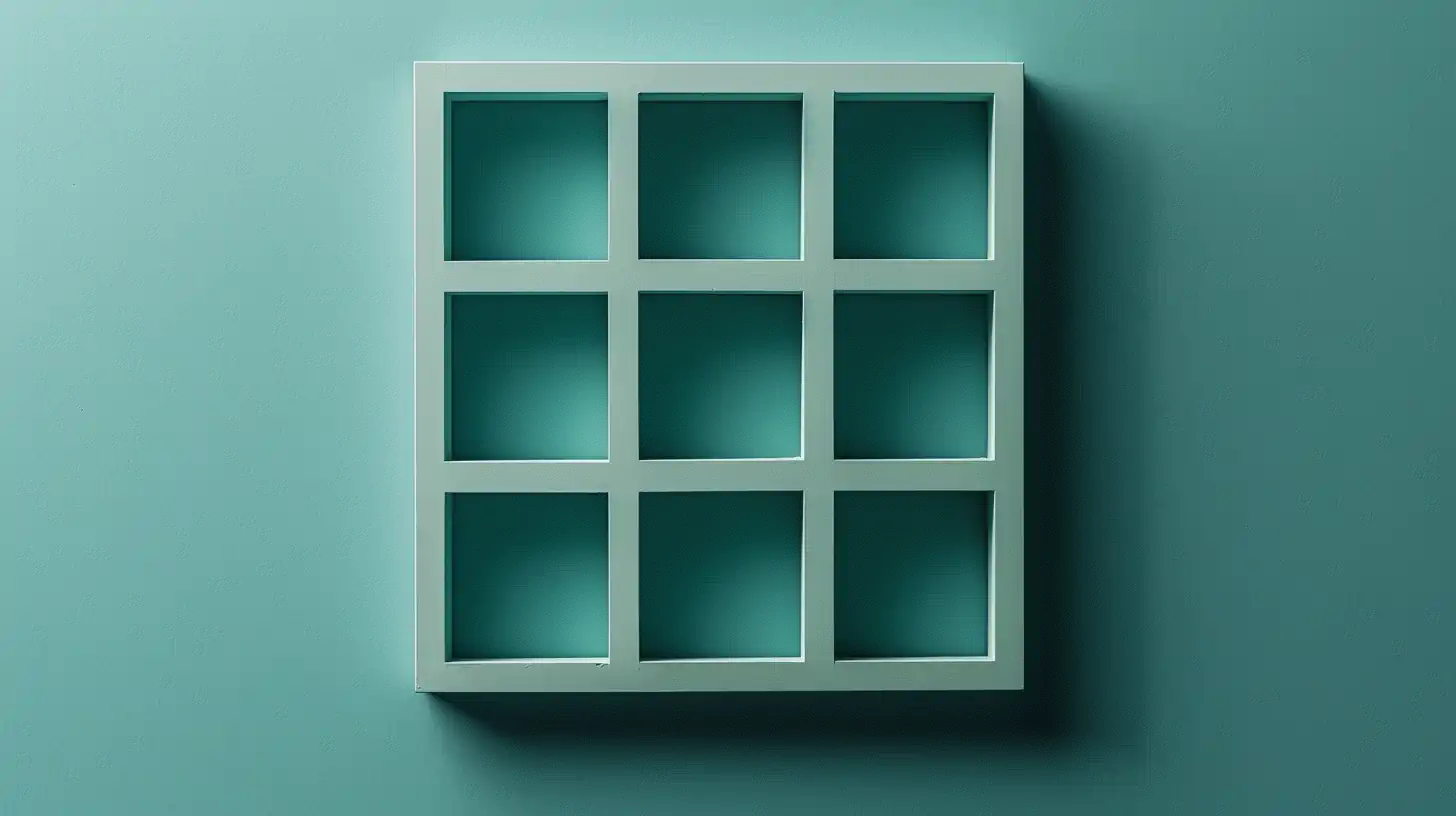
One the greatest advancements in CSS was the introduction of CSS grid. Where in the past developers would have to do grid-style layouts using a combination of CSS floats and clears, now, CSS has a built-in API for creating grid layouts.
While there are a lot of different CSS properties for working with Grid, for simple grids, the three most important rules are:
grid-template-columns
which defines the number of columns in a single row of your grid.column-gap
which defines the horizontal space between columns in a single row of your grid.row-gap
which defines the vertical space between multiple rows of your grid.
To demonstrate the basics of grid layout, let's wire up a responsive 3x3 grid. Our goal will be to "stack" our grid columns on smaller, mobile screens, and then expand to the full 3x3 grid on larger tablet and desktop screens.
In the CodePen above, we've created our responsive 3x3 grid. To do it, we start with some HTML which has a basic structure like this:
<div class="example-grid">
<div class="grid-cell">
<p>Grid Cell #1</p>
</div>
...
</div>
To get a 3x3 grid, we add a total of 9 grid-cell
divs inside of the example-grid
div.
For our CSS, we add some basic browser resets and then start to style up our grid. Our actual grid behavior is entirely controlled by the CSS we apply to the example-grid
div in our markup above.
This is the actual "grid" with all of the nested grid-cell
divs being the grid's "children." First, in order to "turn on" CSS grid behavior, we set our example-grid
div to display: grid
.
Next, because we want to ensure that our grid stacks vertically on smaller screens, we start by applying the grid-template-columns
rule setting it to repeat(1, 1fr)
. Here, the repeat()
function in CSS tells the browser to "repeat" the pattern of displaying 1
column in the unit of 1fr
. Here, fr
stands for "fraction."
When we write repeat(1, 1fr)
we're saying "display a single column with a width relative to 1 fraction or "slice" of the grid." Because we only want a single column on smaller screens, this translates to "make each cell take up the entire width of the grid."
Where things get interesting is down in our media query. Once we've reached a screen width with enough space to support our 3x3 grid (i.e., the content in each cell doesn't break), we want to "turn on" our 3x3 grid.
To do it, inside of our @media screen and (min-width: 768) {}
media query, we redefine our example-grid
style, this time, replacing repeat(1, 1fr)
with repeat(3, 1fr)
. Here, we're saying we want to display 3 columns in our grid, with each column taking up a width relative to 1 slice of the number of columns (i.e., 1/3 of our grid).
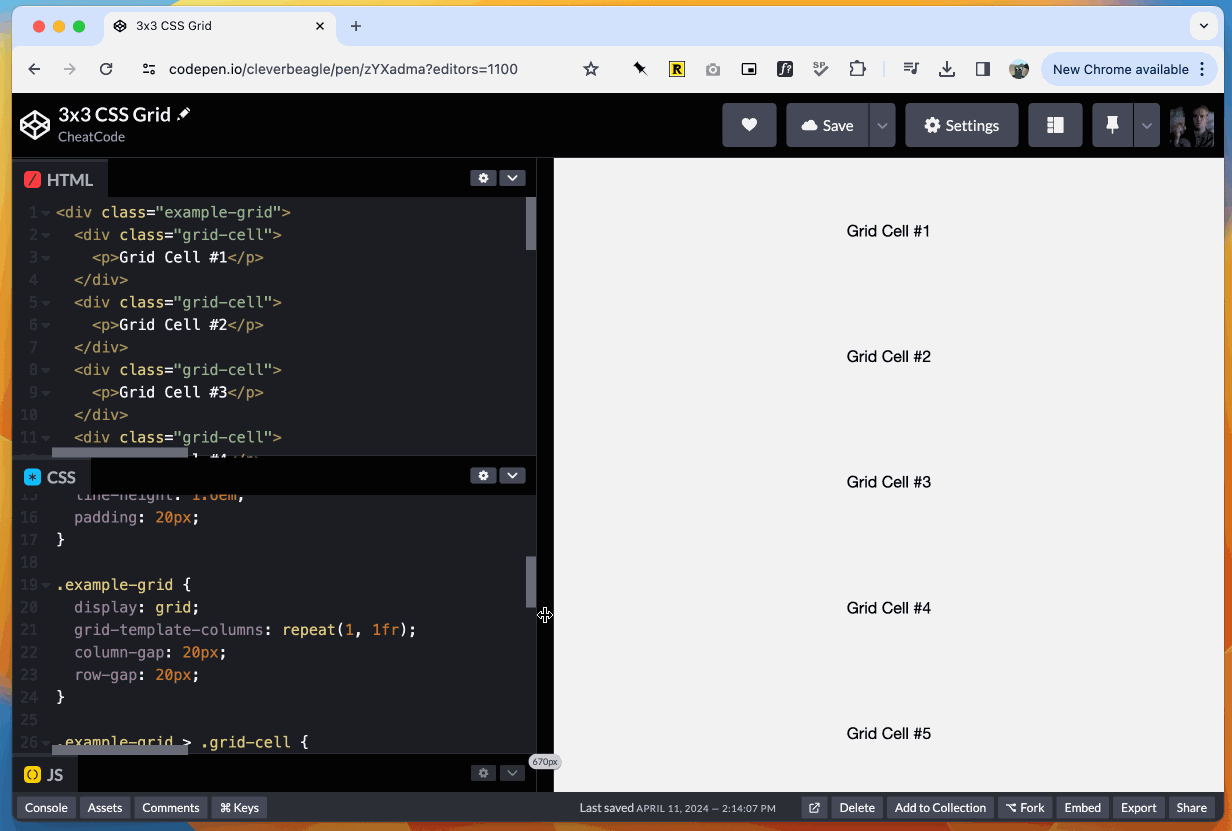
That's it! Now, if we resize our browser from mobile to desktop, we can see our grid expand and collapse accordingly.