tutorial // Mar 10, 2023
How to Insert an Element Into an Array with JavaScript
How to write a function to insert an element into an array at a specific index using JavaScript.
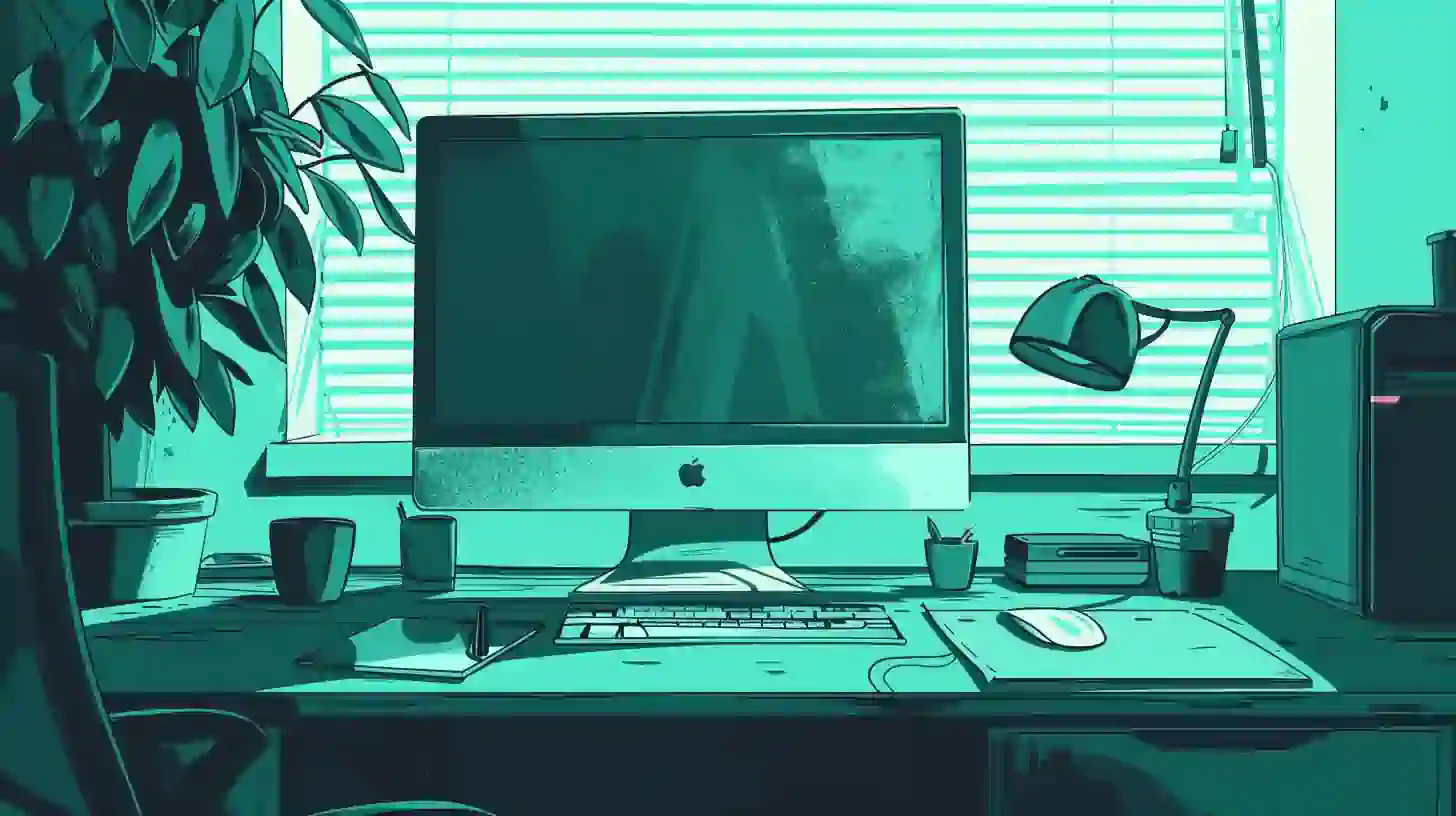
Getting Started
Because the code we're writing for this tutorial is "standalone" (meaning it's not part of a bigger app or project), we're going to create a Node.js project from scratch. If you don't already have Node.js installed on your computer, read this tutorial first and then come back here.
Once you have Node.js installed on your computer, from your projects folder on your computer (e.g., ~/projects
), create a new folder for our work:
Terminal
mkdir app
Next, cd
into that directory and create an index.js
file (this is where we'll write our code for the tutorial):
Terminal
cd app && touch index.js
One last step: in the package.json
file that was created for you, make sure to add the field "type": "module"
as a property. This will enable ES Modules support and allow us to use the import
statements shown in the code below.
With that in place, we're ready to get started.
Writing a function for inserting items
To make our work reusable throughout our app, we're going to add a separate file in the project we just created at /insertInArray.js
:
/insertInArray.js
export default (array = [], index = 0, items = []) => {
// We'll handle the insert here...
};
To start, here, we're creating a skeleton for our function, which will accept three arguments:
array
which will be the array we want to insert items into.index
which is the index or "spot" where we want to insert the item at.items
which is the array of items (one or more) that will be inserted into thearray
.
/insertInArray.js
export default (array = [], index = 0, items = []) => {
const arrayToModify = [...array];
arrayToModify.splice(index, 0, ...items);
return arrayToModify;
};
Next, to do the insert, we're going to leverage the native JavaScript array.splice()
method. This allows us to "splice in" items into our array at a given index. By default, the .splice()
method will modify the original array in memory as opposed to creating a copy of it. Above, we start by creating a copy of the array manually, and storing it in the the variable arrayToModify
to avoid this.
Set to that variable, we're creating a new array []
and using the JavaScript ...
spread operator to "spread out" or unpack the contents of array
into our new array. By doing this, we create a new array that's a 1:1 clone of the original array
.
Next, we call the .splice()
method on our copy arrayToModify
, passing three things:
- The
index
in the array that we want to insert ouritems
at. - How many elements to delete starting at
index
. Here, we use0
because we don't want to delete any items, we just want to add them. - The actual
items
we want to insert into the array.
For the third argument, we use the ...
spread operator to "unpack" the items
array into this place. Because the splice function assumes that every argument after the first two is an item we want to splice/insert into the array. Here, assuming we had an array like [1, 2, 3, 4, 5]
for items
we'd effectively be writing something like .splice(index, 0, 1, 2, 3, 4, 5)
. This—obviously—is more condensed and makes it easy to pass an arbitrary number of items to insert (1 or more).
Finally, once we've done our insert, we return the arrayToModify
expecting it to be updated with our items.
Testing out inserts
That takes care of the core work. Now, let's test out a few usage scenarios to see how this works.
/index.js
import insertInArray from "./insertInArray.js";
const testArray = [1, 2, 3, 4, 5];
console.log('Insert at start', insertInArray(testArray, 0, [6, 7, 8]));
console.log('Insert at middle', insertInArray(testArray, 2, [6, 7, 8]));
console.log('Insert at end', insertInArray(testArray, testArray.length, [6, 7, 8]));
Up at the top, we import our insertInArray
function (notice that we're intentionally adding .js
on the end because we're not using a build tool and Node.js will throw an error without this). Next, we create a testArray
with some numbers. Keep in mind: this can be any array of elements (e.g., strings, objects, etc.). Finally, at the bottom, we have three different calls to console.log()
, passing the location of our insert and then the test case.
That's it! If we run our code with node index.js
, we should see our modified arrays.
Wrapping up
In this tutorial, we learned how to write a function to help us insert items in an array at a given index. We learned how to create a copy of an array to avoid modifying the original using the JavaScript spread operator, and then, how to use the JavaScript array.splice()
method to modify our array. Finally, we looked at some test cases to give us an idea of how to use our function in our app.