tutorial // Mar 31, 2021
How to Install Node.js and Manage Versions with NVM
How to install Node.js and NPM on your computer and how to manage different versions using NVM (Node Version Manager).
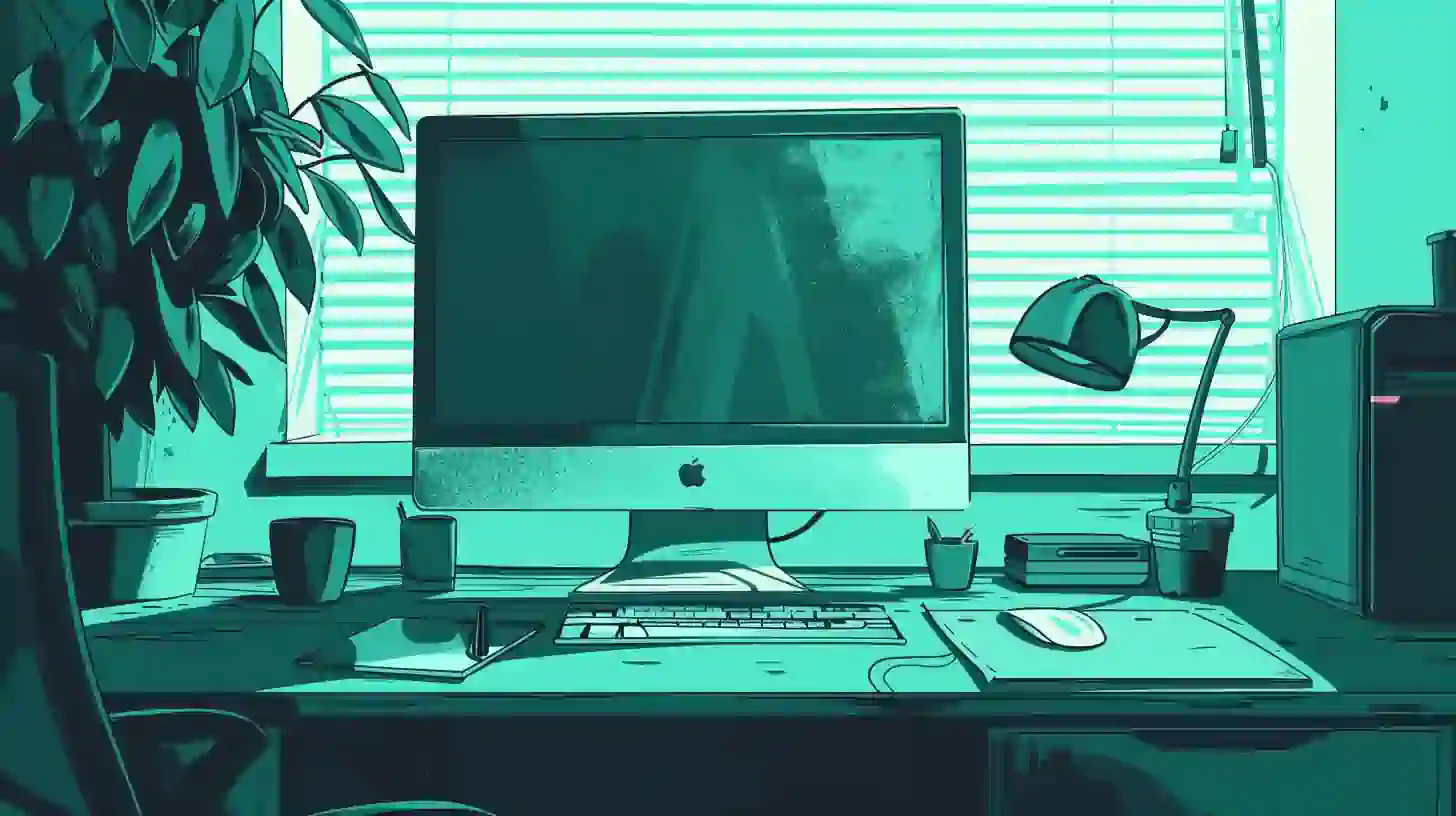
Before you start working with Node.js, you need to have both the Node.js runtime and NPM (Node Package Manager) installed on your computer. By default, only one version of Node.js can be used at a time. If you're working with a wide range of code (e.g., various projects for work, reading tutorials, or completing a course), this can cause issues when Node.js versions don't match.
To fix this, it's best to install the NVM (Node Version Manager) command line tool to help you quickly and easily switch between Node.js versions on your computer.
To get started, first we'll take a look at installing Node.js the traditional way (and the difference between the versions available), and then we'll see how NVM can help us to manage different versions of Node.js.
Installing Node.js LTS vs. Current Version
The easiest way to install Node.js is to head over to the Node.js website and download the installer for your operating system. On that page, you'll be presented with two options:
X.X.X LTS
- The current "Long Term Support" version of the runtime. This is considered the current "stable" version of the runtime and is ideal for projects where maximum compatibility and stability is required.X.X.X Current
- The latest version of the runtime. This is the most recent iteration of the runtime and is ideal for projects that want to leverage the latest Node.js features, or, for developers who don't mind working around unpatched issues.
Though the "LTS" version is denoted as being "Recommended for Most Users," using the current version helps to reduce confusion when reading tutorials, completing courses, and using third-party tools based on Node.js.
Recommendation: if this is your first time using Node.js, install the "LTS" version until you get comfortable with the runtime and then install the current version to get access to the current full feature set.
Managing Versions of Node.js with NVM
As you progress with Node.js, you will find that different packages (like those found on NPM), different tutorials, and different courses will use different versions of Node.js.
If you only follow the instructions above and install a single version of Node.js on your computer, this means that if some code was written that only works with an older or newer version of Node.js: you'll run into problems.
While it's not currently possible to automatically switch between Node.js versions, tools like NVM (Node Version Manager) can help. NVM is an unofficial command line tool that helps you to easily switch between Node.js versions on your computer.
For example, with NVM installed, if we're currently using Node.js v15.13.0
but the code we're working with requires v8.0.0
, with NVM we can run:
nvm install 8
and then have version 8 of Node.js automatically downloaded, installed, and set as the active Node.js version on our computer.
Installing NVM (Node Version Manager)
To install NVM, head over to the project's repo on Github. As the instructions explain there, to install NVM we'll need to use the command line program curl
to download NVM onto our computer.
To do that, on a Mac, open up your Terminal or on Windows your Command Line and run the following:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.38.0/install.sh | bash
Note: Be mindful of the /v0.38.0
part here. That is the current version as of writing and may have changed since this post was last updated.
After you run this, the script at that URL will download the necessary dependencies for NVM and install it on your computer. After it completes, you will see a message about closing and re-opening your terminal to start using NVM.
First, close your terminal (close the entire app to be safe as we need to force our terminal to reload). Next, before you restart it, locate your terminal's profile on your computer.
Your terminal or command line profile includes default settings and code necessary to run your terminal. This code be third-party programs (like NVM) or configuration for the terminal itself.
For MacOS users, this is located at the root of your computer in your user directory (e.g., ~/rglover
). To locate it, open up a Finder window and click on your username in the left-hand navigation. In that folder, look for a file called .bash_profile
, .zshrc
, .profile
, or .bashrc
(this will vary based on your computer's setup but .bash_profile
is most common) and open that file in a text editor (e.g., Visual Studio Code).
Note: For MacOS users, if you're having trouble finding the file, you may need to turn on hidden files in Finder. To do it, open your terminal back up and run:
defaults write com.apple.finder AppleShowAllFiles YES
killall Finder
This will force hidden files to be shown in Finder and then restart Finder with killall Finder
. After this is complete, go ahead and close your terminal again (completely close the app).
Once you've located the file, scroll to the bottom and place the script starting with the export
keyword from the NVM docs and paste it at the bottom of the file:
export NVM_DIR="$([ -z "${XDG_CONFIG_HOME-}" ] && printf %s "${HOME}/.nvm" || printf %s "${XDG_CONFIG_HOME}/nvm")"
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh" # This loads nvm
As the comment on the end suggests, this code is responsible for loading NVM into your terminal on startup. Keep in mind, as a convenience the NVM installer does attempt to add this code for you so it may already be added to the file.
Note: for Windows users, this may be different depending on which shell environment you're using.
After that's complete, you should be ready to start using NVM.
Using NVM to Manage Node.js Versions
With NVM installed, open your terminal back up and then run nvm
. You should see the manual for NVM printed into your terminal. From here, you can start to install and manage different versions of Node.js using the commands listed.
~ $ nvm
Node Version Manager
Note: <version> refers to any version-like string nvm understands. This includes:
- full or partial version numbers, starting with an optional "v" (0.10, v0.1.2, v1)
- default (built-in) aliases: node, stable, unstable, iojs, system
- custom aliases you define with `nvm alias foo`
Any options that produce colorized output should respect the `--no-colors` option.
Usage:
[...]
The important commands to know are:
nvm install X.X.X
This command helps you to install a specific version of Node.js. You can type out the full 3-digit semantic version for any existing Node.js version, or if you're not sure or don't care, you can type nvm install X
where X
is a major version to install the latest patch version of that major release (e.g., if we run nvm instal 15
, as of writing, we'll get v15.13.0
installed).
nvm use X.X.X
This command helps you to switch between your already installed versions of Node.js. For example, if we previously ran nvm install 15
to install the latest major version of Node.js but now we need to switch to Node.js version 8.15.1
for an older project, we can run nvm use 8
and NVM will automatically switch the Node.js version in our terminal to the latest patch version of Node.js 8.
nvm uninstall X.X.X
This command helps you to remove previously installed versions of Node.js using NVM. While you technically don't need to do this, removing unused or rarely used versions of Node.js can help to free up space on your computer.
How NPM Packages Behave When Using NVM
One gotcha with using NVM is that when you switch between versions, you may have issues with certain dependencies. For example, if we've installed the webpack
NPM package on our computer, the version of that package may depend on a different Node.js version than what we're currently using.
This means that when we run the webpack
command (included as part of that package), we may get an ambiguous error that some feature that was expected to exist does not:
~ $ nvm use 4
Now using node v4.6.2 (npm v2.15.11)
~ $ webpack
/Users/rglover/.nvm/versions/node/v4.6.2/lib/node_modules/webpack/bin/webpack.js:85
let packageManager;
^^^
SyntaxError: Block-scoped declarations (let, const, function, class) not yet supported outside strict mode
at exports.runInThisContext (vm.js:53:16)
at Module._compile (module.js:373:25)
at Object.Module._extensions..js (module.js:416:10)
at Module.load (module.js:343:32)
at Function.Module._load (module.js:300:12)
at Function.Module.runMain (module.js:441:10)
at startup (node.js:139:18)
at node.js:990:3
The error presented here may not make sense out of context, but the issue is that the version of webpack
installed in our global NPM packages requires a later version of Node.js than what NVM has us currently using (here, v4.6.2
).
If this happens when trying to run your own project, it's best to start by switching to the latest version of Node.js available (or installing it first if you haven't already) and then trying to run the command again.
If that doesn't work, it's best to go and research the package in question a bit further and see if its documentation specifies any Node.js version required for it to work.
Wrapping Up
Using NVM alongside Node.js is a great way to improve your development workflow and streamline your day-to-day work with Node.js. It's best utilized by developers who work across a range of Node.js projects, or, are still in the process of learning and moving between different tutorials and courses on the internet.